import numpy as np
from itertools import permutations
def is_in_gershgorin_disks(matrix):
# Check if all eigenvalues of the matrix are in the Gershgorin disks
eigenvalues = np.linalg.eigvals(matrix)
n = matrix.shape[0]
for i in range(n):
center = matrix[i, i]
radius = np.sum(np.abs(matrix[i, :])) - np.abs(center)
if not np.all(np.abs(eigenvalues - center) <= radius):
return False
return True
def permute_rows(matrix, permutation):
# Permute rows in the matrix based on the given permutation
return matrix[list(permutation), :]
def monte_carlo_approximation(matrix, num_samples=1000):
n = matrix.shape[0]
for _ in range(num_samples):
# Randomly sample a permutation
permutation = np.random.permutation(n)
# Permute rows
permuted_matrix = permute_rows(matrix, permutation)
# Check if the permuted matrix satisfies Gershgorin's Circle Theorem
if is_in_gershgorin_disks(permuted_matrix):
return permutation, permuted_matrix
return None, None
# Input matrix
original_matrix = np.array([
[0, 0, 1],
[1, 0, 0],
[0, 1, 0]
])
# Monte Carlo approximation
permutation, permuted_matrix = monte_carlo_approximation(original_matrix, num_samples=1000)
if permutation is not None:
print(f"\nRows permutation {permutation} results in a matrix satisfying Gershgorin's Circle Theorem:")
print(permuted_matrix)
else:
print("No permutation found in the Monte Carlo approximation.")
dijous, 28 de desembre del 2023
Gershgorin with montecarlo
Gershgorin Circle Theorem
I want to get the matrice where all ones are next to diagonal.
Seems it could be get from
import numpy as np |
dimecres, 27 de desembre del 2023
row sorting
import numpy as np
matrix = np.array([
[0, 1, 0, 0, 0],
[0, 0, 0, 1, 0],
[1, 0, 0, 0, 0],
[0, 0, 1, 0, 0],
[0, 0, 0, 0, 1]
])
num_rows = matrix.shape[0]
identity = np.eye(num_rows)
for i in range(len(matrix)):
for j in range(len(identity)):
product = matrix[i] * identity[j]
if np.any(product > 0):
print(f"{i+1} > {j+1}")
dilluns, 20 de novembre del 2023
al Moodle, llista d'activitats "no ocultes per als estudiants" i el seu enllaç al Clipboard
function copyToClipboard(data) {
const el = document.createElement('textarea');
el.value = data.map(row => row.join('\t')).join('\n');
document.body.appendChild(el);
el.select();
document.execCommand('copy');
document.body.removeChild(el);
console.log('Data copied to clipboard.');
}
var xpath = '//li[@class and not(.//*[@class="badge badge-pill badge-warning"])]/.//span[@class="inplaceeditable inplaceeditable-text"]/a[@href]';
var elements = document.evaluate(xpath, document, null, XPathResult.ORDERED_NODE_SNAPSHOT_TYPE, null);
var linksList = [];
for (var i = 0; i < elements.snapshotLength; i++) {
var linkElement = elements.snapshotItem(i);
var linkText = linkElement.textContent.trim();
var linkHref = linkElement.getAttribute('href');
// Check if linkText or linkHref contains #
if (linkText.includes('#') || (linkHref && linkHref.includes('#'))) {
// Skip this iteration if # is present
continue;
}
linksList.push([linkText, linkHref]);
}
copyToClipboard(linksList);
divendres, 22 de setembre del 2023
Programa pel ChromeBook
per accedir a Snippets i poder entrar el programa al navegador Chrome:
- premem F12
- Ctrl+Majúsc+P
- Snippet
- New snippet
const row = [];
for (let multiplier = 1; multiplier <= 10; multiplier++) {
row.push(i * multiplier);
}
console.log(row.join('\t'));
}
el resultat és:
dimarts, 12 de setembre del 2023
dilluns, 11 de setembre del 2023
Criteris d'avaluació - a l'excel
Criteris d'avaluació - del codi a la descripció
dijous, 7 de setembre del 2023
Chrome Webdriver (solved from August 2023)
divendres, 25 d’agost del 2023
Excel - Label text linked to cells
Dim counter As Integer
Sub InsertCellTextIntoTextbox()
counter = counter + 1
Dim ws As Worksheet
Set ws = ActiveSheet
Dim xCoord As Double
Dim yCoord As Double
' Get the coordinates of the active cell
xCoord = ActiveCell.Left
yCoord = ActiveCell.Top
Dim shp As Shape
Set shp = ws.Shapes.AddTextbox(Orientation:=msoTextOrientationHorizontal, _
Left:=xCoord, Top:=yCoord, Width:=100, Height:=30)
' Get the text from the cell corresponding to the counter value
shp.TextFrame.Characters.Text = ws.Cells(counter, 1).Value
shp.TextFrame.Characters.Font.Size = 16
shp.TextFrame.Characters.Font.Bold = True
End Sub
Sub AssignShortcutKey()
Application.OnKey "^+B", "InsertCellTextIntoTextbox" ' Ctrl + Shift + B
End Sub
Excel - Label insert When Ctrl+Shift+B
Dim counter As Integer
Sub InsertIncrementedLabel()
counter = counter + 1
Dim ws As Worksheet
Set ws = ActiveSheet
Dim xCoord As Double
Dim yCoord As Double
' Get the coordinates of the active cell
xCoord = ActiveCell.Left
yCoord = ActiveCell.Top
Dim shp As Shape
Set shp = ws.Shapes.AddTextbox(Orientation:=msoTextOrientationHorizontal, _
Left:=xCoord, Top:=yCoord, Width:=100, Height:=30)
shp.TextFrame.Characters.Text = "Label " & counter
shp.TextFrame.Characters.Font.Size = 16
shp.TextFrame.Characters.Font.Bold = True
End Sub
Sub AssignShortcutKey()
Application.OnKey "^+B", "InsertIncrementedLabel" ' Ctrl + Shift + B
End Sub
dijous, 27 de juliol del 2023
dimecres, 26 de juliol del 2023
Levenstein a EXCEL
Function Levenshtein(ByVal string1 As String, ByVal string2 As String) As Long
Dim i As Long, j As Long
Dim string1_length As Long
Dim string2_length As Long
Dim distance() As Long
string1_length = Len(string1)
string2_length = Len(string2)
ReDim distance(string1_length, string2_length)
For i = 0 To string1_length
distance(i, 0) = i
Next
For j = 0 To string2_length
distance(0, j) = j
Next
For i = 1 To string1_length
For j = 1 To string2_length
If Asc(Mid$(string1, i, 1)) = Asc(Mid$(string2, j, 1)) Then
distance(i, j) = distance(i - 1, j - 1)
Else
distance(i, j) = Application.WorksheetFunction.Min _
(distance(i - 1, j) + 1, _
distance(i, j - 1) + 1, _
distance(i - 1, j - 1) + 1)
End If
Next
Next
Levenshtein = distance(string1_length, string2_length)
End Function
dilluns, 24 de juliol del 2023
Drag a txt file to Chrome. Two columns in txt file, tab separated, have values to Levenstein comparison (all from 1st col to all 2nd col)
// Function to calculate Levenshtein distance between two strings
function levenshteinDistance(str1, str2) {
// Create a 2D array to store the distances
const dp = Array.from({ length: str1.length + 1 }, () => Array(str2.length + 1).fill(0));
// Initialize the array with base cases
for (let i = 0; i <= str1.length; i++) {
for (let j = 0; j <= str2.length; j++) {
if (i === 0) dp[i][j] = j;
else if (j === 0) dp[i][j] = i;
else {
const cost = str1[i - 1] === str2[j - 1] ? 0 : 1;
dp[i][j] = Math.min(dp[i - 1][j] + 1, dp[i][j - 1] + 1, dp[i - 1][j - 1] + cost);
}
}
}
return dp[str1.length][str2.length];
}
// Main function to calculate Levenshtein distances and copy results to clipboard
function calculateLevenshteinDistances() {
// Get the content of the current tab
const result = document.evaluate('/html/body/pre', document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null);
const preElement = result.singleNodeValue;
const content = preElement ? preElement.textContent.trim() : '';
const rows = content.split('\n');
// Assuming the headers are present in the first row, you can extract them like this:
const [header1, header2] = rows.shift().split('\t');
// Use the Clipboard API to copy the header row to clipboard
let clipboardData = `${header1}\t${header2}\tLevenshteinDistancesCalculated\n`;
// Use the Clipboard API to append each row of data to clipboard
for (let i = 0; i < rows.length; i++) {
const [column1Value, column2Value] = rows[i].split('\t');
if (column1Value !== '-' && column2Value !== '-') {
for (let j = 0; j < rows.length; j++) {
const [column1ValueToCompare, column2ValueToCompare] = rows[j].split('\t');
if (column1ValueToCompare !== '-' && column2ValueToCompare !== '-') {
const distance = levenshteinDistance(column1Value, column2ValueToCompare);
clipboardData += `${column1Value}\t${column2ValueToCompare}\t${distance}\n`;
}
}
}
// Send a progress message to the console after each row is processed
console.log(`Processing row ${i + 1} of ${rows.length}`);
}
// Copy the final clipboardData to the clipboard
copyToClipboard(clipboardData);
// Send completion message to the console
console.log('Levenshtein distance calculations completed.');
}
// Function to copy text to clipboard
function copyToClipboard(text) {
const el = document.createElement('textarea');
el.value = text;
document.body.appendChild(el);
el.select();
document.execCommand('copy');
document.body.removeChild(el);
console.log('Data copied to clipboard.');
}
// Call the function to execute the code
calculateLevenshteinDistances();
dilluns, 17 de juliol del 2023
Get a list of unique words sorted alphabetically from drag txt file
const result = document.evaluate('/html/body/pre', document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null);
const preElement = result.singleNodeValue;
const content = preElement ? preElement.textContent.trim() : '';
// Split the content into an array of words
const words = content.split(/\s+/);
// Create a Set to store unique words
const uniqueWords = new Set(words);
// Convert the Set back to an array and sort it alphabetically
const sortedWords = Array.from(uniqueWords).sort();
console.log('Content:', content);
console.log('Word Count:', words.length);
console.log('Unique Words:', sortedWords);
// Open a new tab with the sorted words
const newTab = window.open('');
newTab.document.write('<html><head>');
newTab.document.write('<style>body { font-family: Consolas; font-size: 10pt; }</style>');
newTab.document.write('</head><body>');
newTab.document.write('<h1>Sorted Words</h1>');
newTab.document.write('<ul>');
sortedWords.forEach((word) => {
newTab.document.write(`<li>${word}</li>`);
});
newTab.document.write('</ul>');
newTab.document.write('</body></html>');
newTab.document.close();
Drag the txt file in Chrome and refer to it as Content object
const result = document.evaluate('/html/body/pre', document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null);
const preElement = result.singleNodeValue;
const content = preElement ? preElement.textContent.trim() : '';
console.log('Content:', content);
dimecres, 21 de juny del 2023
dilluns, 12 de juny del 2023
Variaciones con repetición 2 elementos para cuatro espacios
function generateCombinations(gaps, currentCombination = '') {
if (gaps === 0) {
return currentCombination + '<br>';
}
const combinations = [];
combinations.push(...generateCombinations(gaps - 1, currentCombination + 'C'));
combinations.push(...generateCombinations(gaps - 1, currentCombination + '+'));
return combinations;
}
// Generate combinations for 4 gaps
const combinations = generateCombinations(4);
// Open a new window with the results
const newWindow = window.open();
newWindow.document.write('<html><body>');
newWindow.document.write('<pre>' + combinations.join('') + '</pre>');
newWindow.document.write('</body></html>');
newWindow.document.close();
divendres, 9 de juny del 2023
X Y pointer position on Chrome
// Create the rectangle element
var rectangle = document.createElement("div");
rectangle.style.position = "fixed";
rectangle.style.top = "50px";
rectangle.style.left = "50px";
rectangle.style.width = "200px";
rectangle.style.height = "100px";
rectangle.style.backgroundColor = "rgba(0, 0, 0, 0.5)";
rectangle.style.color = "#fff";
rectangle.style.padding = "10px";
rectangle.style.fontFamily = "Arial";
rectangle.style.fontSize = "16px";
rectangle.style.zIndex = "9999";
// Append the rectangle to the document body
document.body.appendChild(rectangle);
// Update the mouse position values in the rectangle
document.onmousemove = function(e) {
var position = {
x: e.clientX,
y: e.clientY
};
rectangle.innerHTML = "Mouse Position: X = " + position.x + ", Y = " + position.y;
};
dimarts, 6 de juny del 2023
parágrafos del REBT que coinciden con una palabra "motor", se incluyen el parágrafo previo y el posterior, así como título
//https://www.boe.es/buscar/act.php?id=BOE-A-2002-18099
function copyToClipboard(text) {
const el = document.createElement('textarea');
el.value = text;
document.body.appendChild(el);
el.select();
document.execCommand('copy');
document.body.removeChild(el);
console.log('Data copied to clipboard.');
}
var headers = document.querySelectorAll('h3, h4, h5');
var plainText = '';
for (var i = 0; i < headers.length; i++) {
var header = headers[i];
var correspondingParagraph = header.nextElementSibling;
var codeBefore = '';
var codeAfter = '';
while (correspondingParagraph) {
if (
correspondingParagraph.nodeName === 'P' &&
correspondingParagraph.classList.length > 0 &&
correspondingParagraph.textContent.includes('motor')
) {
var prevSibling = correspondingParagraph.previousElementSibling;
var nextSibling = correspondingParagraph.nextElementSibling;
if (prevSibling && prevSibling.tagName === 'P') {
codeBefore = prevSibling.textContent;
}
if (nextSibling && nextSibling.tagName === 'P') {
codeAfter = nextSibling.textContent;
}
plainText += header.textContent + ':\n';
plainText += codeBefore + '\n';
plainText += correspondingParagraph.textContent + '\n';
plainText += codeAfter + '\n';
break;
}
correspondingParagraph = correspondingParagraph.nextElementSibling;
}
}
copyToClipboard(plainText);
capítulos del REBT 842/2002 en los que se menciona "tierra" > to be run as prompt in Console DevTools
//https://www.boe.es/buscar/act.php?id=BOE-A-2002-18099
function copyToClipboard(text) {
const el = document.createElement('textarea');
el.value = text;
document.body.appendChild(el);
el.select();
document.execCommand('copy');
document.body.removeChild(el);
console.log('Data copied to clipboard.');
}
var headers = document.querySelectorAll('h3, h4, h5');
var plainText = '';
for (var i = 0; i < headers.length; i++) {
var header = headers[i];
var correspondingParagraph = header.nextElementSibling;
while (correspondingParagraph) {
if (
correspondingParagraph.nodeName === 'P' &&
correspondingParagraph.classList.length > 0 &&
correspondingParagraph.textContent.includes('tierra')
) {
plainText += header.textContent + ': ' + correspondingParagraph.textContent + '\n';
break;
}
correspondingParagraph = correspondingParagraph.nextElementSibling;
}
}
copyToClipboard(plainText);
dimecres, 24 de maig del 2023
List of labels whose checkmark is checked. Copy them in clipboard
(function() {
var checkboxes = document.querySelectorAll('input[type="checkbox"]');
var checkedLabels = [];
checkboxes.forEach(function(checkbox) {
if (checkbox.checked) {
var label = checkbox.nextElementSibling;
if (label && label.innerText) {
checkedLabels.push(label.innerText);
}
}
});
// Copy the checked labels to the clipboard
var textToCopy = checkedLabels.join('\n');
var tempInput = document.createElement("textarea");
document.body.appendChild(tempInput);
tempInput.value = textToCopy;
tempInput.select();
document.execCommand("copy");
document.body.removeChild(tempInput);
console.log("Labels of checked checkboxes copied to clipboard.");
})();
Checkbox marked as string in a text label - DevTools in Chrome Snippet
(function() {
var checkboxes = document.querySelectorAll('input[type="checkbox"]');
checkboxes.forEach(function(checkbox) {
var label = checkbox.nextElementSibling;
if (label && label.innerText.includes(")*_")) {
checkbox.checked = true;
}
});
})();
dissabte, 13 de maig del 2023
dijous, 11 de maig del 2023
dimecres, 26 d’abril del 2023
SQL amb IIf imbricats Mid i InStr
SELECT Mid(Sheet1.Field1,InStr(Sheet1.Field1,"; Di")+2,InStr(Sheet1.Field1,"h.;")-InStr(Sheet1.Field1,"; Di")) AS DiaHora, Mid(Sheet1.Field1,InStr(Sheet1.Field1,"; del "),InStr(Sheet1.Field1,"; Di")-InStr(Sheet1.Field1,"; del ")) AS DataData, Mid(Sheet1.Field1,InStr(Sheet1.Field1,"_M"),5) AS MP_UF_,
IIf(InStr([Sheet1].[Field1], "IEA") > 0,
IIf( Mid([Sheet1].[Field1],InStr([Sheet1].[Field1],"IEA"),3)="IEA","IEA"),
IIf(InStr([Sheet1].[Field1], "GAD") > 0,
IIf( Mid([Sheet1].[Field1],InStr([Sheet1].[Field1],"GAD"),3)="GAD","GAD"),
IIf(InStr([Sheet1].[Field1], "TAPSD") > 0,
IIf( Mid([Sheet1].[Field1],InStr([Sheet1].[Field1],"TAPSD"),3)="TAPSD","TAPSD"),
IIf(InStr([Sheet1].[Field1], "AFI") > 0,
IIf( Mid([Sheet1].[Field1],InStr([Sheet1].[Field1],"AFI"),3)="AFI","AFI"),
IIf(InStr([Sheet1].[Field1], "EDI") > 0,
IIf( Mid([Sheet1].[Field1],InStr([Sheet1].[Field1],"EDI"),3)="EDI","EDI"),"")))))
AS Cicle1
FROM Sheet1;
divendres, 21 d’abril del 2023
clic a un conjunto de botones a través de su xpath - DevTools Console
const elements = document.evaluate('//a[@class="answer flex items-center text-xl text-aac-orange hover:no-underline"]', document, null, XPathResult.ORDERED_NODE_SNAPSHOT_TYPE, null);
for (let i = 0; i < elements.snapshotLength; i++) {
const element = elements.snapshotItem(i);
element.click();
}
dijous, 6 d’abril del 2023
Open the Device Manager from the Run dialog box (Win+R)
You can open the Device Manager from the Run dialog box (Win+R) by following these steps:
Press Win+R to open the Run dialog box.
Type "devmgmt.msc" (without the quotes) in the "Open" field and press Enter or click OK.
This will open the Device Manager window where you can view and manage the devices installed on your computer.
dimarts, 4 d’abril del 2023
List of available cameras on computer
Get-CimInstance -ClassName Win32_PnpEntity | Where-Object {$_.Caption -like '*camera*'} | Select-Object Name, Caption
PowerTip: Use PowerShell to Discover Laptop Webcam
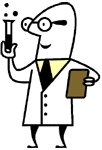
Doctor Scripto
Summary: Use Windows PowerShell to discover a webcam attached to your laptop.
How can I use Windows PowerShell to find a webcam or camera that is attached to my laptop?
Use the Get-CimInstance or the Get-WmiObject cmdlet, examine the Win32_PnpEntity WMI class,
and look for something that matches camera in the caption.
By using Get-CimInstance in Windows PowerShell 3.0 or later:
Get-CimInstance Win32_PnPEntity | where caption -match 'camera'
By using Get-WmiObject in Windows PowerShell 2.0:
Get-WmiObject Win32_PnPEntity | where {$_.caption -match 'camera'}
Note Not all webcams populate exactly the same way, so it may take a bit of searching
to find the right string.